In the world, it is very common to create objects or modify or add specific components to objects.
ZEPETOScript supports the same interface as that used in the Unity script.
Basic Examples of GameObject and Component
Creating and removing game objects:
// GameObject Create
const tempObj = new GameObject();
const obj = Object.Instantiate(tempObj);
// GameObject Destroy
Object.Destroy(obj);
To get GameObjects components or add new ones, use GetComponent or AddComponent.
// GetComponent with Generic
const myTransform = this.GetComponent<Transform>();
// AddComponent with Generic
const animator = this.gameObject.AddComponent<Animator>();
Check the full code example for the GameObject Component.
Here's an example that covers the creation and property modification of GameObject, addition of components, and destruction.
import { GameObject, Object, Transform, Animator, Vector3, WaitForSeconds } from 'UnityEngine';
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
export default class GameObjectSample extends ZepetoScriptBehaviour {
private _testObject : GameObject;
Start() {
// Create an empty GameObject
const tempObj = new GameObject();
this._testObject = Object.Instantiate(tempObj) as GameObject;
// Change the object's name
this._testObject.name = "TestObject";
// Change the object's transform information
this._testObject.transform.position = new Vector3(3,3,3);
// Attach a component to the object
this._testObject.gameObject.AddComponent<Animator>();
// Example of using GetComponent
const animator = this._testObject.GetComponent<Animator>();
if(animator != null) {
console.log("GetComponent Success");
}
// Destroy the object after 5 seconds using a coroutine
this.StartCoroutine(this.DestroyObject());
}
*DestroyObject() {
yield new WaitForSeconds(5);
// Destroy the GameObject
Object.Destroy(this._testObject);
}
}
Utilizing Find
The Find related methods also support the same interface style in ZEPETOScript as in Unity script.
Methods | Description |
---|---|
GameObject.Find() | - It finds and returns an active GameObject object based on the name in the current Scene. - Returns null if not found. |
GameObject.FindGameObjectWithTag() | - It finds and returns an active GameObject object based on the tag in the current Scene. - Finds and returns the first object matching the specified tag among the active objects, returns null if not found. |
GameObject.FindGameObjectsWithTag() | - Finds all active GameObject objects with a specific tag in the current Scene and returns them as an array. - Returns an empty array if none are found. |
For the example, set up the Scene as follows:
- Add several 3D objects and specify all their Tags as 3D.
- Create an Empty Object to attach the script to, and rename it to FindSample.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { GameObject, Transform } from 'UnityEngine';
export default class FindSample extends ZepetoScriptBehaviour {
public tempObj1: GameObject;
public tempObj2: GameObject;
public tempObj3: GameObject[];
Start() {
// Finds the target GameObject with the specified name.
// If multiple GameObjects have the same name, the first one found will be returned.
this.tempObj1 = GameObject.Find("Cube");
if(this.tempObj1 != null) {
console.log("GameObject Find Success");
}
// Finds the target GameObject with the specified tag.
// If multiple GameObjects have the same tag, the first one found will be returned.
this.tempObj2 = GameObject.FindGameObjectWithTag("3D");
if(this.tempObj2 != null) {
console.log("FindGameObjectWithTag Success");
}
// Returns an array of GameObjects that have the specified tag.
this.tempObj3 = GameObject.FindGameObjectsWithTag("3D");
if(this.tempObj3 != null) {
console.log("FindGameObjectsWithTag Success");
}
}
}
- The inspector is empty when you add a script in the FindSample object.
- Press the Play button to run it, and you can confirm in the Inspector window that each object has been assigned and check the success of finding objects through the console window.
Creating Prefabs
Let's explore how to create prefabs during runtime.
- First, add a 3D object > Cube to the Scene.
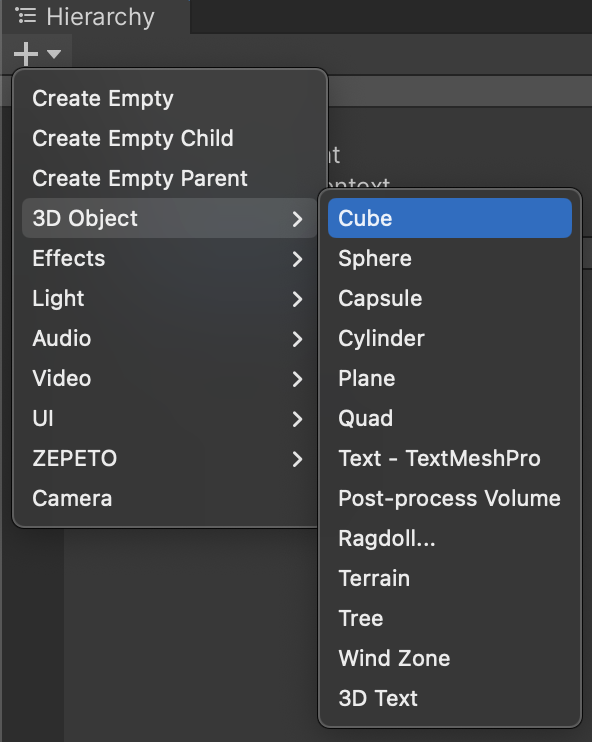
- Press Add Component in the inspector of Cube, and add Rigidbody.
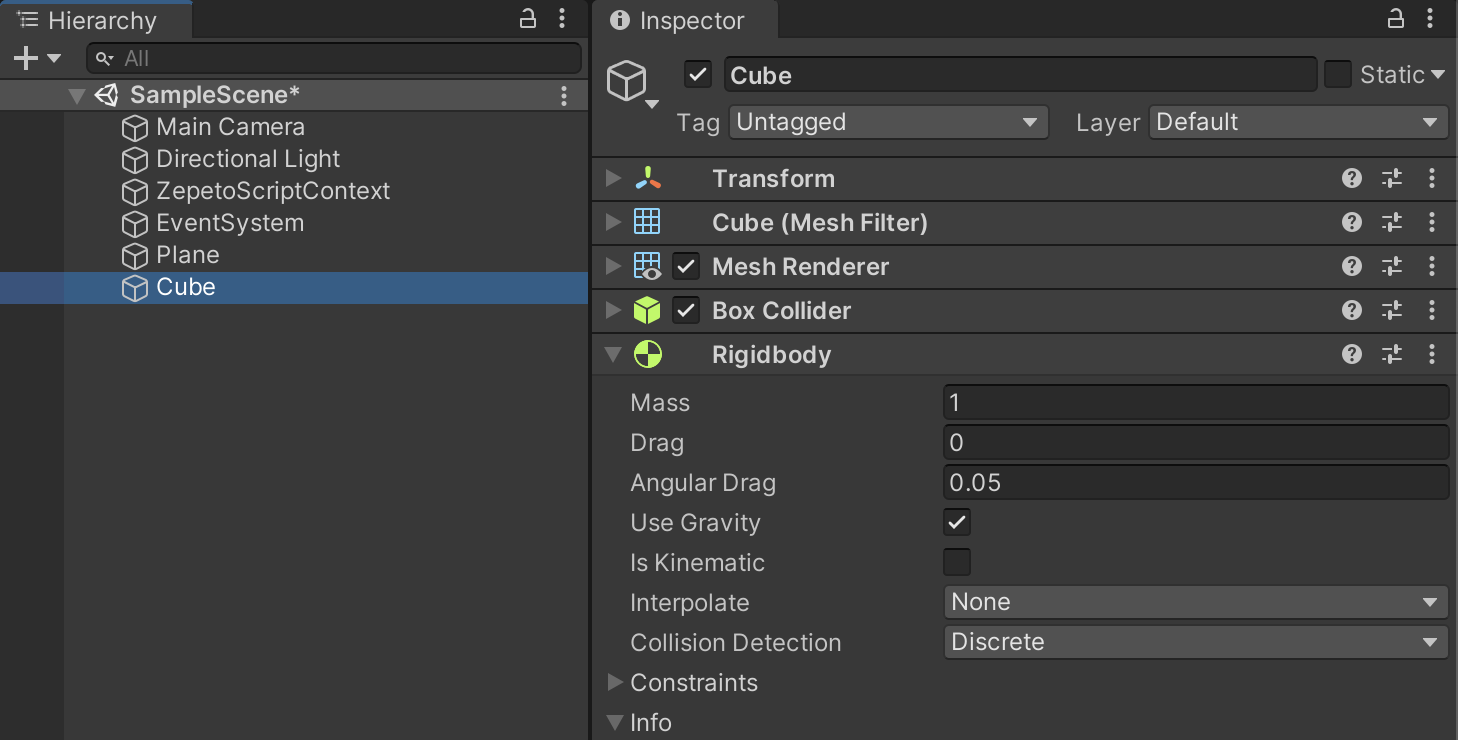
- Then drag the Cube to the project area to make it a prefab.
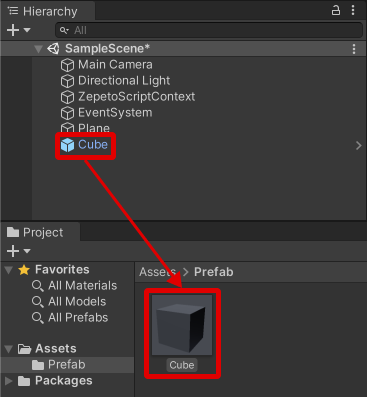
- Since we will utilize the Prefab, delete the Cube in the Scene.
- Please write the script below.
import { GameObject, Object, Vector3, WaitForSeconds } from 'UnityEngine';
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
export default class CloneSample extends ZepetoScriptBehaviour {
public clonePrefab : GameObject;
Start() {
this.StartCoroutine(this.DoRoutine());
}
*DoRoutine() {
while(true) {
yield null;
// Create a clone
const clone = Object.Instantiate(this.clonePrefab) as GameObject;
// Set the height of the clone object
clone.transform.position = new Vector3(0, 10, 0);
// Destroy it after 5 seconds of creation
GameObject.Destroy(clone, 5);
yield new WaitForSeconds(1);
}
}
}
- Then return to the Unity editor, drag the prefab to the script inspector to add it.
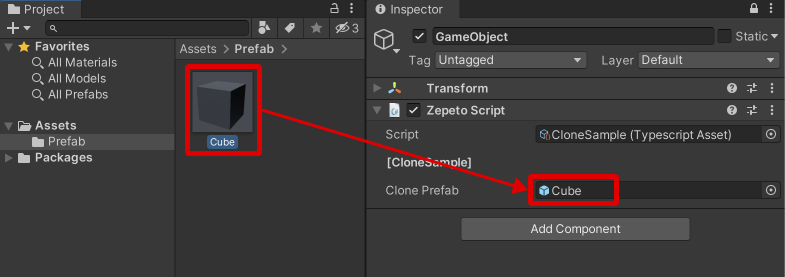
- Press the play button to check that a 3D Object is created and falls from above every second.
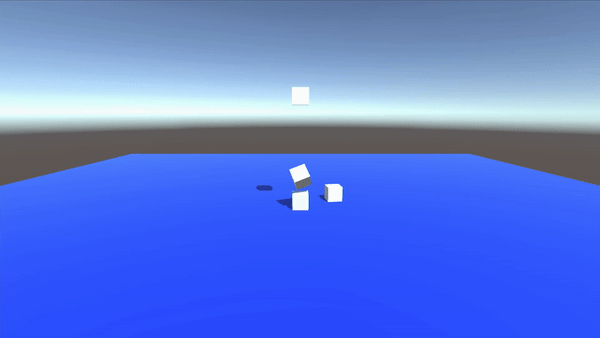
Click the links below to learn more about the Unity GameObjects and Components offered by ZEPETOScript.
Creating GameObjects
https://docs.unity3d.com/2020.3/Documentation/ScriptReference/Object.Instantiate.html
Destroying GameObjects
https://docs.unity3d.com/2020.3/Documentation/ScriptReference/Object.Destroy.html
Using components
https://docs.unity3d.com/kr/current/Manual/UsingComponents.html