CREATE YOUR WORLD
Players & Characters: Tips
Controlling ZEPETO camera fixation
4min
The ZEPETO camera is set to follow the player's ZEPETO character. This guide will show you how to prevent the ZEPETO camera from following your ZEPETO character at runtime.
- Add a GameObject to the Scene and include the RuntimeCamera example script below.
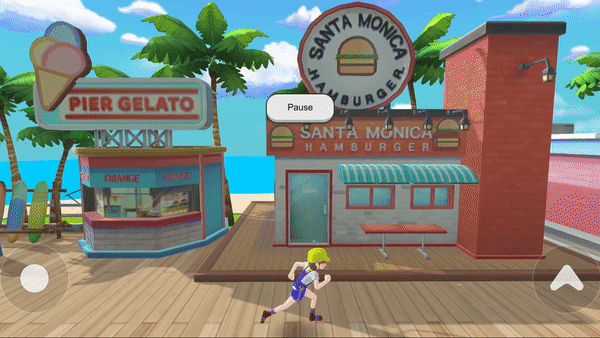
- If you press the Pause button, you will notice that the ZEPETO camera no longer follows the player character and remains fixed in place.
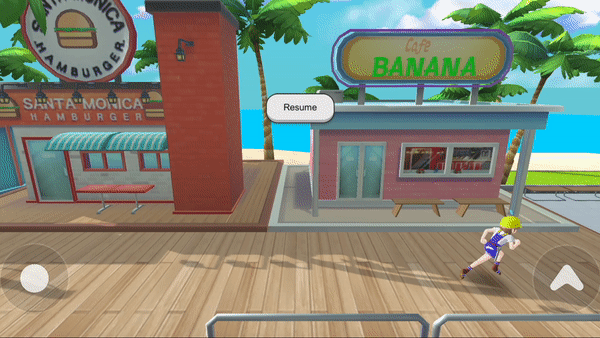
- When you press the Resume button, you can see that the camera follows the character again.
Updated 10 Oct 2024

Did this page help you?