Gesture
ZepetoWorldContent API allows you to set thumbnails for desired gesture/pose categories and enable specific gestures/poses when a thumbnail is clicked.
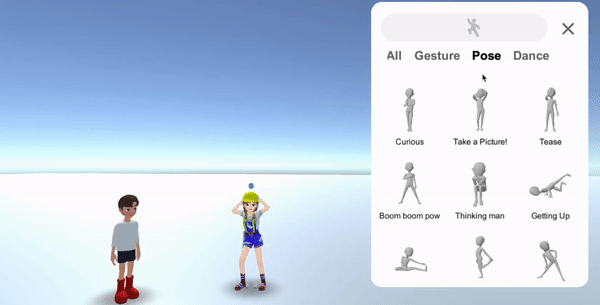
To use the ZepetoWorldContent API, you must write an import statement as follows.
The member variable and function information of the Content class containing gesture/pose information are as follows:
API | Description |
---|---|
public get Id(): string | Content Unique Id |
public get Title(): string | Gesture, pose title text - The language will automatically translate depending on device language |
public get Thumbnail(): UnityEngine.Texture2D | 2D thumbnail |
public get AnimationClip(): UnityEngine.AnimationClip | Gesture Animation Clip |
public get IsDownloadedThumbnail(): boolean | Function to determine if you have previously downloaded this thumbnail |
public get IsDownloadedAnimation(): boolean | Function to determine if you have previously downloaded this animation clip |
public DownloadAnimation($complete: System.Action):void | An animation clip download function that receives a completed callback with a factor - If IsDownloadedAnimation() is false, implement DownloadAnimation() to be called. |
public DownloadThumbnail($complete: System.Action):void | Function to download thumbnails - If IsDownloadedThumbnail() is false, implement DownloadThumbnail() to be called. |
OfficialContentType : enum | Type of content (World 1.9.0 and higher) - Gesture = 2 - Pose = 4 - Selfie = 8 - GestureGreeting = 16 - GesturePose = 32 - GestureAffirmation = 64 - GestureDancing = 128 - GestureDenial = 256 - GestureEtc = 512 - All = 14 |
- You can use the existing function, public DownloadThumbnail($character: ZEPETO_Character_Controller.ZepetoCharacter, $complete: System.Action):void, without any issues regarding functionality. However, since it no longer accepts Zepeto character as an argument, please use the newly modified function public DownloadThumbnail($complete: System.Action):void instead.
1) Add Hierarchy > UI > Canvas and set Sort Order to 2 to avoid being obscured by other UI.

2) Add Hierachy > UI > Button.

1) Add Hierarchy > Create Empty Object and rename it PanelParent.

2) Add Hierarchy > UI > Panel as a child of PanelParent.

3) Close button: After adding UI > Button, add onClick event to disable gesture panel.

4) Open button : Please add an onClick event that activates the gesture panel to the open button created above.

5) Add an image to serve as the title area.

6) Configure a scroll view to display the gesture thumbnail.
- Add Hierarchy > UI > Scroll View.
- Check off Horizontal and disable the scroll bar image since you will only use vertical scrolls and horizontal scrolls will not be required.
- Add Grid layout to Content in Scroll View to align thumbnails in grid pattern.
- Add Content Size Fitter to make the size of the object appropriate for the size of the content.
- When you implement a script, you must set the content in Scroll View as the parent of the gesture thumbnail (so that the entire area is recognized and scrolled)

7) Configure tabs by gesture type.
- Add Hierarchy > Create Empty Object as a child oft the Panel and rename it to GestureTitle.
- This is the parent object of the toggle button.
- Add Horizontal Layout to align tabs horizontally.
- Add the Toggle Group component.
👍 To configure more tabs, add Hierarchy > UI > Scroll View and check Horizontal in the Scroll View option.

8) Add the text to be used as the toggle button as the child of the GestureTitle, and replace it with All.
- Sets the color of the text to gray.
- Add the highlighted text that will be displayed when checked as a child of the text.
- Set the font content, size, and thickness the same, and set the color to black.
- Add the Toggle component.
- Specify the parent object in the group.
- Add highlighted text that you added as a child to Graphic
- Check isOn only for the All toggle components that will be shown first.
- Create both the Gesture and Pose toggle buttons in the same way.

Use the method of creating a thumbnail button as a pre-fab and then generating it as an instance in a script.
1) Add UI > Button as a child of Content in Scroll View and rename it preThumb.
2) Please change the name to Thumb after adding Raw Image.
- This image will be a thumbnail. Adjust the size appropriately.
3) Add Text.
- Set the position to center the bottom of the image.
- Adjust the size and thickness of the writing, and add the Content Size Fitter.
- Horizontal Fit : Preferred Size
- Vertical Fit : Preferred Size
4) If the setting is done, please make it a Prefab and put it in the Resources folder.


👍 UI size and position values shown in the video are recommended, but you can modify them to the values you want!
Once the UI setup is complete, proceed to Scripting.
This script is based on single play.
- Project > Create > ZEPETO > TypeScript and rename it Thumbnail.
- Write a sample script like below.
- This is a script that organizes gesture content information (title, image) into the UI.
- After creating the script, open the preThumb prefab and add the script.

- Create a Hierarchy > Create Empty Object and rename it to GestureLoader.
- Create a Project > Create > ZEPETO > TypeScript and rename it to GestureLoader.
- Write a sample script like below.
- Count is the maximum number of gestures to download on each tab. If you set it to a number larger than 100, there may be errors during the thumbnail download process, so please set it only as much as you need.
The script flows as follows:
1) Invoke the ContentsRequest() custom function for generating thumbnails after loading the ZEPETO character.
- The ContentsRequest() function receives content information by separating gestures and poses, respectively.
- If there is an existing thumbnail, it is skipped; otherwise, the thumbnail is retrieved.
- The retrieved thumbnail data is stored in respective lists.
2) The CreateThumbnailObject() custom function is then called.
- The CreateThumbnailObject() function takes information from the thumbnail list to create a thumbnail button prefab instance.
- In the Thumbnail script within the prefab, set the Thumbnail information of the content to the thumbnail button image, and set the Title information of the content to the thumbnail button text. Apply the generated thumbnail button to the UI panel.
- Click the thumbnail button to call the LoadAnimation custom function and play the corresponding gesture using the SetGesture() function.
- Assign Content Parent, Thumbnail Prefab, and Count to the Inspector after completing the script.
- The entry in Contents Parent is the Content in Scroll View.

- Create a Hierarchy > Create Empty Object and rename it to UIContoller.
- Create Project > Create > ZEPETO > TypeScript and rename it to UIContoller.
- Write a sample script like below.
The script flows as follows:
- Touch the touchpad or close button to cancel the play using the CancelGesture() function.
- Tap the tab (toggle button) to invoke the SetCategoryUI() custom function.
- The SetCategoryUI() function uses the gesture content information in each thumbnail to set it for each corresponding category.
- Enable if it's an applicable type, and disable if not.
After completing the scripting, assign Close Button, TypeToggleGroup to the Inspector.
- The entry to the Type Toggle Group is the Toggle that is a child of the Toggle Group in the gesture panel.

❗️ Caution Before playing, disable PanelParent so that only the open button can be seen when playing.

- In the case of multi-play, a synchronization code must be added that receives the gesture information value taken by a particular player and applies it to all players accessing the Room.
- The key is to send and receive a Room Message between the server and the client about which player made which gesture.
- Write the same script as the one implemented in the single-play client code.
- By default, scripts implemented in single-play client code are written the same.
- Additionally, the client declares the interface to contain the PlayerGestureInfo.
- When sending your information to the server: see SendMyGesture() Custom Function
- When your player presses the thumbnail to make a gesture, send the gesture ID to the server using room.Send().
- When you cancel a gesture, process it to send the information that you canceled.
- When receiving gesture information from another client from the server: "OnChangeGesture" Room Message is sent to this.room.AddMessageHandler() within Start()
- Synchronization is achieved by having the session ID and gesture ID in the "OnChangeGesture" message and making the appropriate player play the gesture.
- After completing the scripting, the Inspector will assign an additional object to Multiplay with the Zepeto World Multiplay component.


- By default, scripts implemented in single-play client code are written the same.
- The difference from single-play client code is the StopGesture() custom function.
- Invoke a SendMyGesture() custom function within the GestureLoaderMultiplay.
- Process to send information that the gesture has been canceled.
- The server code then declares the interface to contain the PlayerGestureInfo in the same way.
- The server code is based on the server code in the Multiplay Sample by default.
- It creates an onMessage() callback that sends gesture information to other clients when a gesture changes within onCreate().
