Change local player costume
You can change a local player’s outfit to the ones that you own.
ZEPETO.Character.Controller 1.11.3 or higher version must be installed.
API | Description |
---|---|
SetCostume($itemCode: string, $complete?: System.Action):void; | Enter the item code (item id) as the argument value to change the local player’s outfit. Receive a callback once the outfit change is complete. |
You can change the local player's costume using SetCostume().
Here is an example code that creates a local player when the scene starts, and changes the outfit with a specific item code when the local player is created.
1) Add ZEPETO > Typescript and name the script ChangeLocalPlayerCostume.
Write a sample script as below.
- This script changes the outfit of the local player according to the given item code.
- When the scene starts, create a player with the specified user ID and use the CreatePlayerWithUserId() function.
- An OnAddedLocalPlayer event listener is added so that when a local player is added, that event is triggered, and the ChangeCostume() method is called to change the costume.
- Access the local player instance through the LocalPlayer property and change the costumes using the provided item code. Once the costume change is complete, a Set Costume Complete message will be logged, indicating a successful change.
📘 Please refer to the mannequin guide on how to check the item id. [ZEPETO Mannequin]
2) If you press the [▶︎(play)] button to run it, you can see that the local player is created and changed with the costume based on entered item code.

Before the costume change (left), after the costume change (right)
- If the item is a fixed-term product, it will be worn after checking whether it has expired. For items that have expired, an error log called Expired item is called.
- If the item is a Zem paid product, it will be worn after checking whether it is owned by a local player. For items not owned, an error log called Has Not ItemCode is called.
You can retrieve the list of clothing items by utilizing GetMyItemListAsync(), which provides information about the items owned by the user.
The following example demonstrates how to display the outfits owned by a local player on the screen and enable them to select and change outfits.
First, create a UI list resource where the thumbnail and name of the clothing list will be displayed. Resources that need to be crafted include:
After loading the list of clothes the local player has, we create a list of items in the UI. The Item Prefab serves as a source for each item list being created.
As the Item Prefab is created, the thumbnail and item name are displayed in the UI.
The item Prefab contains the following elements.
- Button_thumbnail object: This is an object where the Raw Image component and Button component are registered to display the thumbnail image of the item.
- Text_name object: A Text component object in which the item name will be displayed.

Canvas is the UI screen displayed when Item Prefab is created. It is recommended to create a Scroll View inside this Canvas so that a large list of items can be retrieved.
The Scroll View object inside the Canvas is set as follows.
- The Scroll View object is set to allow only vertical scrolling.
- The Content object sets up the following components:
- Grid Layout Group:
- CellSize : x: 150, Y: 150
- Constraint : Fixed Column Count
- Constrait Count : 5

- Add ZEPETO > Typescript and rename the script to MyItemList.
- Write a sample script as below.
- In the Start method, a new player is created using ZepetoPlayers.instance.CreatePlayerWithUserId()and the CoGetMyItem() coroutine is executed when the local player is added.
- The CoGetMyItem() coroutine uses ShopService.GetMyContentItemListAsync() to retrieve the list of items owned by the player.
- Request a list of all items in all categories via ItemKeyword.all. Wait until the request is complete using yield new WaitUntil(() => requestItemList.keepWaiting == false).
- If the request is successful requestItemList.responseData.isSuccess, iterates through the list of contentItems and retrieves each item's thumbnail image using GetThumbnailAsync().
- Create a UI element for each item using the provided itemPrefab, and set the thumbnail image and ID. A button for each item is created, and set to call SetItemButton() with the ID of the item as an argument.
- The SetItemButton() method is called when the button associated with an item is clicked.
- Apply the selected item as the player's character costume using ZepetoPlayers.instance.LocalPlayer.SetCostume().
- When the costume is successfully changed, a log message saying Set Costume Complete is displayed along with the item code.
📘 Tips
- Please refer to the following guide on how to check the item information of the user through ShopService.GetMyContentItemListAsync(). [Get information about items owned by a user]
- When searching for items owned by the user, you can search by category using ItemKeyword and display them in a list on the UI.
❗️ Caution The list of clothes retrieved through ShopService.GetMyContentItemListAsync() contains expired time-limited products. So Expired item error log can be called.
Register the itemPrefab created in step 1 in the Item Prefab of the component to which MyItemList is applied, and register the Canvas in the itemCanvas.

If you press the Play button to run it, the canvas UI will display a list of items owned by the local player, and you can see that the item changes to the corresponding outfit when selected.
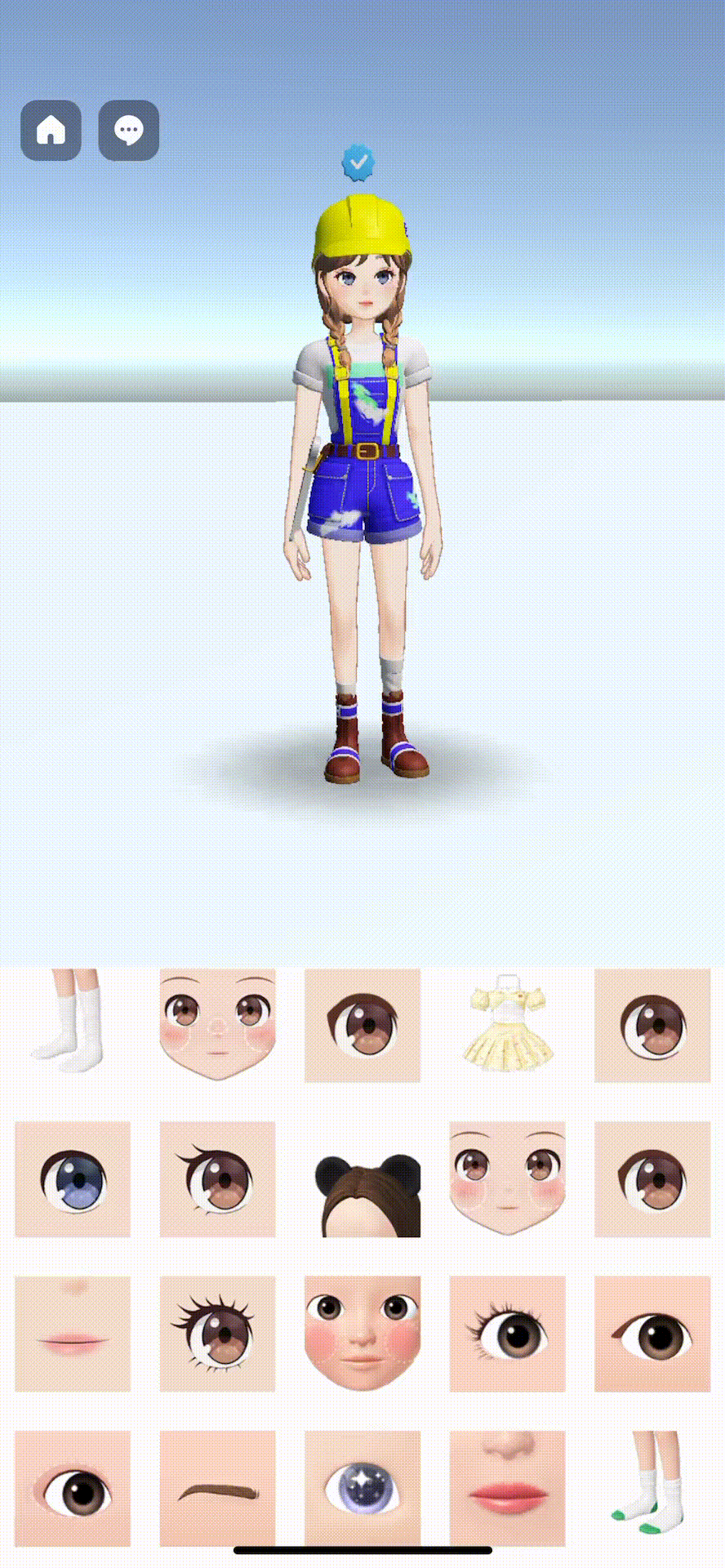
❗️ Caution
- This costume wearing API only applies to local players and does not currently support multiplayer synchronization.
- Costume multiplayer synchronization will be available in the future.

