In this guide, we will show you how to measure the height of a local player's ZEPETO character according to the body type.
STEP 1 : Write a script to get the height value of the character
Add a GameObject to the Scene and add the CharacterHeight example script below.
import { Debug, HumanBodyBones, Vector3, WaitForEndOfFrame } from 'UnityEngine';
import { LocalPlayer, ZepetoPlayers, ZepetoPlayer, KnowSockets } from 'ZEPETO.Character.Controller';
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
export default class CharacterHeight extends ZepetoScriptBehaviour {
Start() {
ZepetoPlayers.instance.OnAddedLocalPlayer.AddListener(() => {
let _player: LocalPlayer = ZepetoPlayers.instance.LocalPlayer;
this.StartCoroutine(this.CoGetZepetoHeight(_player.zepetoPlayer));
});
}
*CoGetZepetoHeight(zepeto: ZepetoPlayer) {
// Use WaitForEndOfFrame to accurately obtain the joint positions of the character.
yield new WaitForEndOfFrame();
// Get the position of the head.
const headPosition = zepeto.character.GetSocket(KnowSockets.HEAD_UPPER).position;
// Get the position of the left foot.
const leftFootPosition = zepeto.character.ZepetoAnimator.GetBoneTransform(HumanBodyBones.LeftFoot).position;
// Get the position of the right foot.
const rightFootPosition = zepeto.character.ZepetoAnimator.GetBoneTransform(HumanBodyBones.RightFoot).position;
// Calculate the midpoint between the two feet.
const characterCenter = Vector3.Lerp(leftFootPosition, rightFootPosition, 0.5);
// Calculate the distance between the head and the character center.
const characterHeight = Vector3.Distance(headPosition, characterCenter);
console.log(`Character Height From Ground: ${characterHeight}`);
}
}
Script Description
*CoGetZepetoHeight(zepeto: ZepetoPlayer)
- Use
GetSocket
to get the character's head socket position value. - To obtain the position value of the bottom center of the character, use
GetBoneTransform
to obtain the position value of both feet of the character. - Use
Vector3.Lerp
to get the character's bottom center position value. - Obtain the character's Height value using the character's head socket and the center bottom position value.
- Use
STEP 2 : Run
When you run it by clicking the Play button, you can see the character's Height value in the console log.
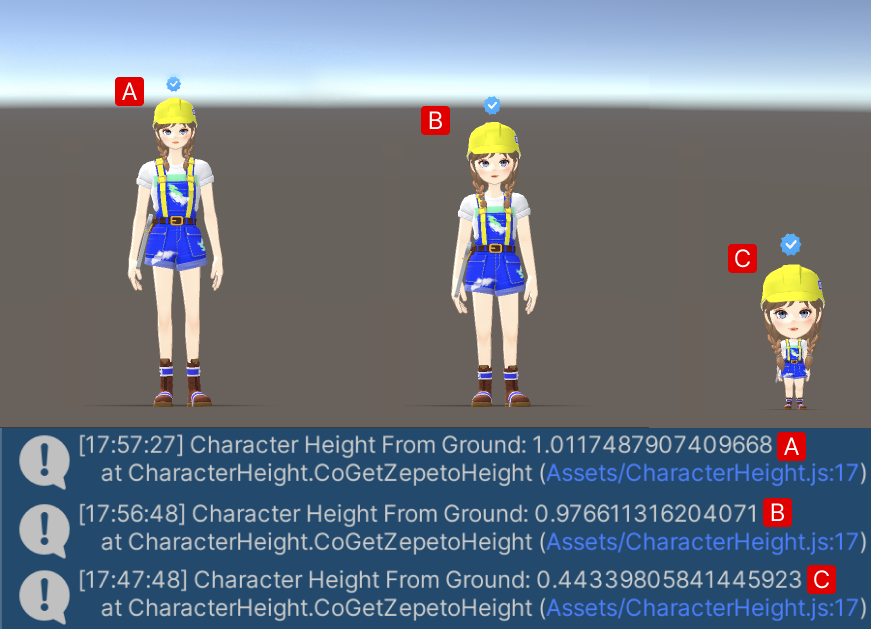
TIP
- The way to measure the height in the example is to measure the character's height using the height value of the HeadSocket.
- If you would like to measure the top position by modeling the character's head, hat, and accessories, please refer to the following link :
SkinnedMeshRenderer.localBounds