In this guide, we will show you how to check if the local player's Zepeto character is on the ground based on the isGrounded
function provided by Unity.
CharacterController.isGrounded
https://docs.unity3d.com/ScriptReference/CharacterController-isGrounded.html
STEP 1 : Write a script
- Add a GameObject to the Scene and add the IsGrounded example script below.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script'
import { ZepetoPlayers } from 'ZEPETO.Character.Controller';
import { Text } from 'UnityEngine.UI';
export default class IsGrounded extends ZepetoScriptBehaviour {
public myText: Text;
Update() {
// Access the local player's character controller
const localCharacter = ZepetoPlayers.instance.LocalPlayer.zepetoPlayer.character.characterController;
// Check if the character controller is touching another collider using isGrounded
if (localCharacter.isGrounded) {
this.myText.text = "Character is on the Ground";
} else {
this.myText.text = "Character is NOT on the Ground";
}
}
}
STEP 2 : Setting up Text UI
- Add UI > Text to the Scene.
- In the Hierachy window, connect the Canvas > Text component to the Property displayed in the ZEPETOScript Inspector window by dragging and dropping.
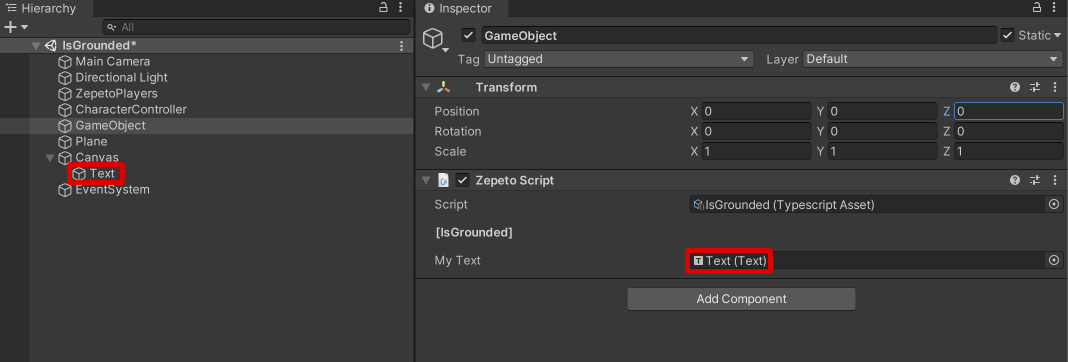
STEP 3 : Run
- If you press the Play button to run it, you can check whether the character is on the floor through the Text UI.
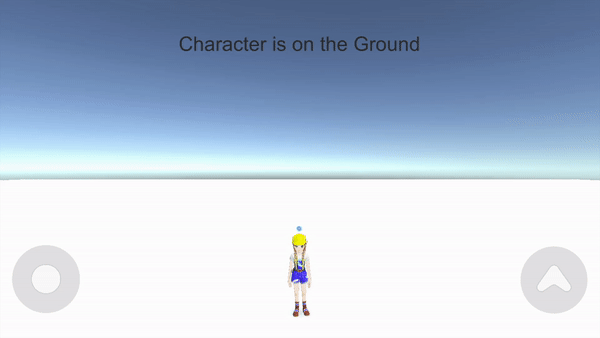