When a ZEPETO avatar entering the world is wearing a body modification item or has chosen an excessively large or small body shape, it can hinder the gameplay in the world.
Using the API introduced in this guide, you can change the ZEPETO character back to its default body shape.
Caution
- The ZEPETO.Character.Controller package is available from version 1.11.1 onwards.
API | Description |
---|---|
GetBodyModifierProperties() | - Returns information about which part of the body the ZEPETO character is wearing a body modification item. |
DeformDefaultProperties() | - This API removes the body modification items worn by the ZEPETO character and changes it back to its default body shape. |
DeformDefaultCostume() | - This API removes the body modification items worn by the ZEPETO character, changes it to its default body shape, and dresses it in the default ZEPETO costume. - It can also be applied at the ZEPETO Players level. In this case, all ZEPETO players entering the multiplayer world will have their body modification items removed and will be dressed in the default ZEPETO costume. |
None = 0, Skin = 1, SkinTone = 2, SkinDetail = 3, Face = 4, Eye = 5, EyeLens = 6, Eyebrow = 7,
Beard = 8, Mustache = 9, EyeShadow = 10, EyeLiner = 11, EyeLash = 12, Blusher = 13, Nose = 14,
Mouth = 15, Lips = 16, Hair = 17, ClothesGlasses = 18, ClothesTop = 19, ClothesBottom = 20, ClothesShoes = 21,
ClothesDress = 22, Background = 23, RoomWallpaper = 24, RoomFloor = 25, RoomBottom = 26, RoomTopLeft = 27, RoomTopRight = 28,
RoomMiddleLeft = 29, RoomMiddleRight = 30, Point = 31, Freckles = 32, FaceHair = 33, DoubleEyelid = 34, NailArt = 35,
ClothesSocks = 36, ClothesGlove = 37, AccessoryBracelet = 38, AccessoryNecklace = 39, AccessoryEarring = 40, AccessoryRing = 41, AccessoryHeadwear = 42,
AccessoryPiercing = 43, BoothBackground = 44, LUT = 45, AccessoryMask = 46, FacePainting = 47, AccessoryBag = 48, AccessoryWing = 49,
ClothesCape = 50, ClothesExtra = 51, MannequinFace = 52, WrinkleForehead = 53, WrinkleEye = 54, WrinkleMouth = 55, DoubleEyelidBottom = 56,
WrinkleMongo = 57, AccessoryTail = 58, AccessoryEffect = 59, ClothesDeform = 60, HairExtensions = 61, MakeupSet = 62, FaceContouring = 63,
BaseModel = 64, CreatorLens = 65, BaseDeform = 66
Example Script
Please refer to the following example script:
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { ZepetoCharacter, LocalPlayer, ZepetoPlayers } from 'ZEPETO.Character.Controller';
import { ZepetoPropertyFlag } from 'Zepeto';
export default class Deform extends ZepetoScriptBehaviour {
private _localPlayer: LocalPlayer;
private _itemProperties : ZepetoPropertyFlag[]
Start() {
ZepetoPlayers.instance.OnAddedLocalPlayer.AddListener(() => {
this._localPlayer = ZepetoPlayers.instance.LocalPlayer;
// 1. Local character - Code to print where the body shape change item is equipped
this._itemProperties = this._localPlayer.zepetoPlayer.character.GetBodyModifierProperties();
console.log(this._itemProperties);
// 2. Local character - code to unequip body shape change item
this._localPlayer.zepetoPlayer.character.DeformDefaultProperties();
// 3. Local character - code to unequip body shape change items and put on default outfit
this._localPlayer.zepetoPlayer.character.DeformDefaultCostume();
});
// 4. Code to unequip all players' body shape change items and put them in default outfits
ZepetoPlayers.instance.OnAddedPlayer.AddListener(() => {
ZepetoPlayers.instance.DeformDefaultCostume();
});
}
}
Script Description
- The body modification item slot information worn by the Local Player is saved in
this._itemProperties
byGetBodyModifierProperties()
and that information is printed to the console
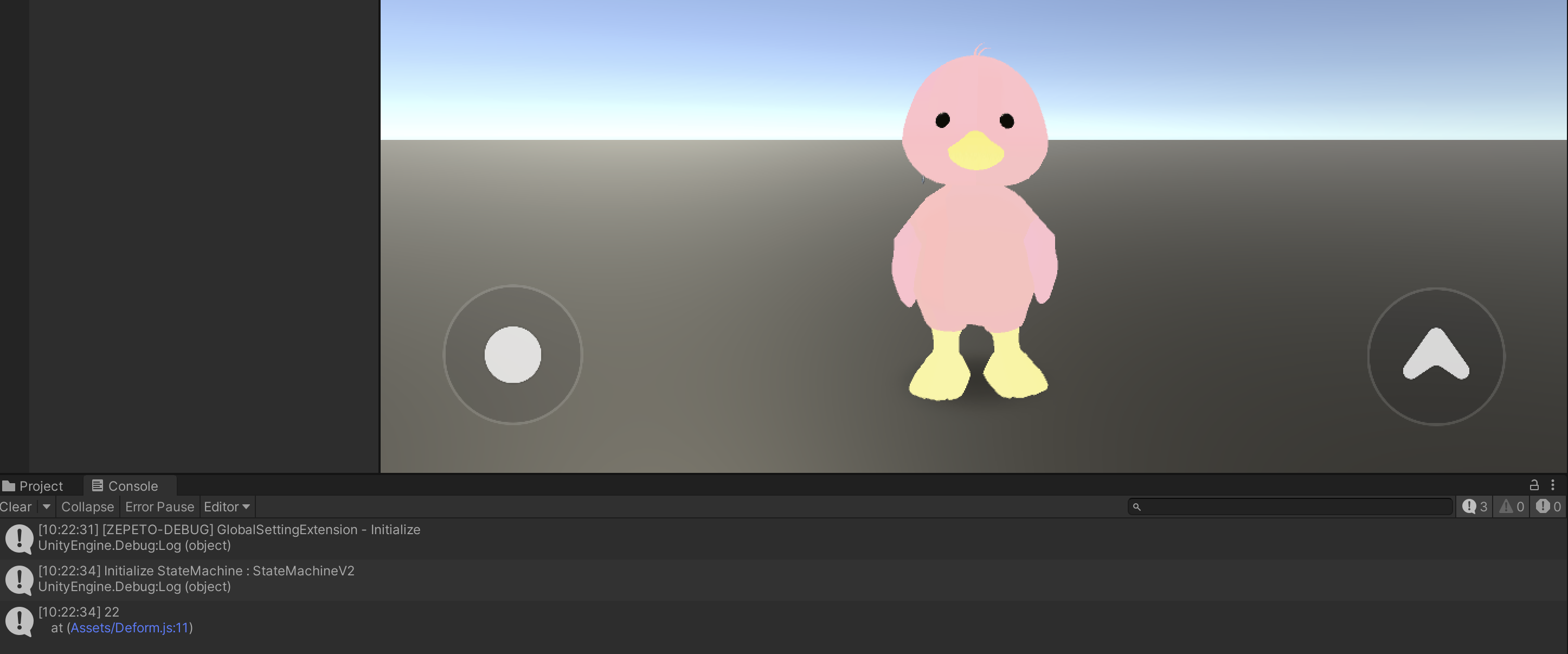
- All body modification items worn by the Local Player are removed by
DeformDefaultProperties()
, and the character is changed back to its default body shape.
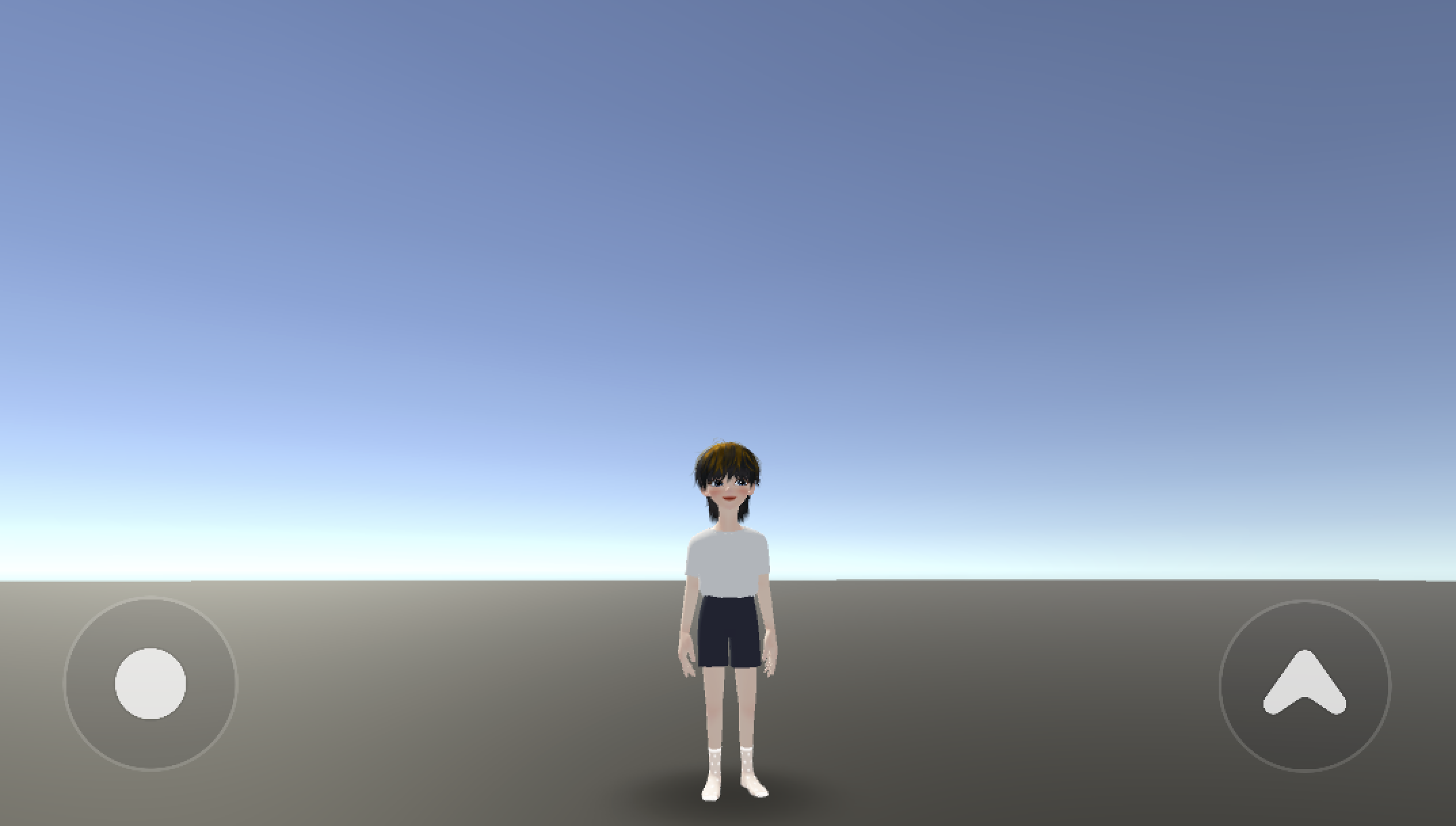
- By
DeformDefaultCostume()
, all body modification items worn by the Local Player are removed, and they are changed to the appearance of wearing the default ZEPETO costume.
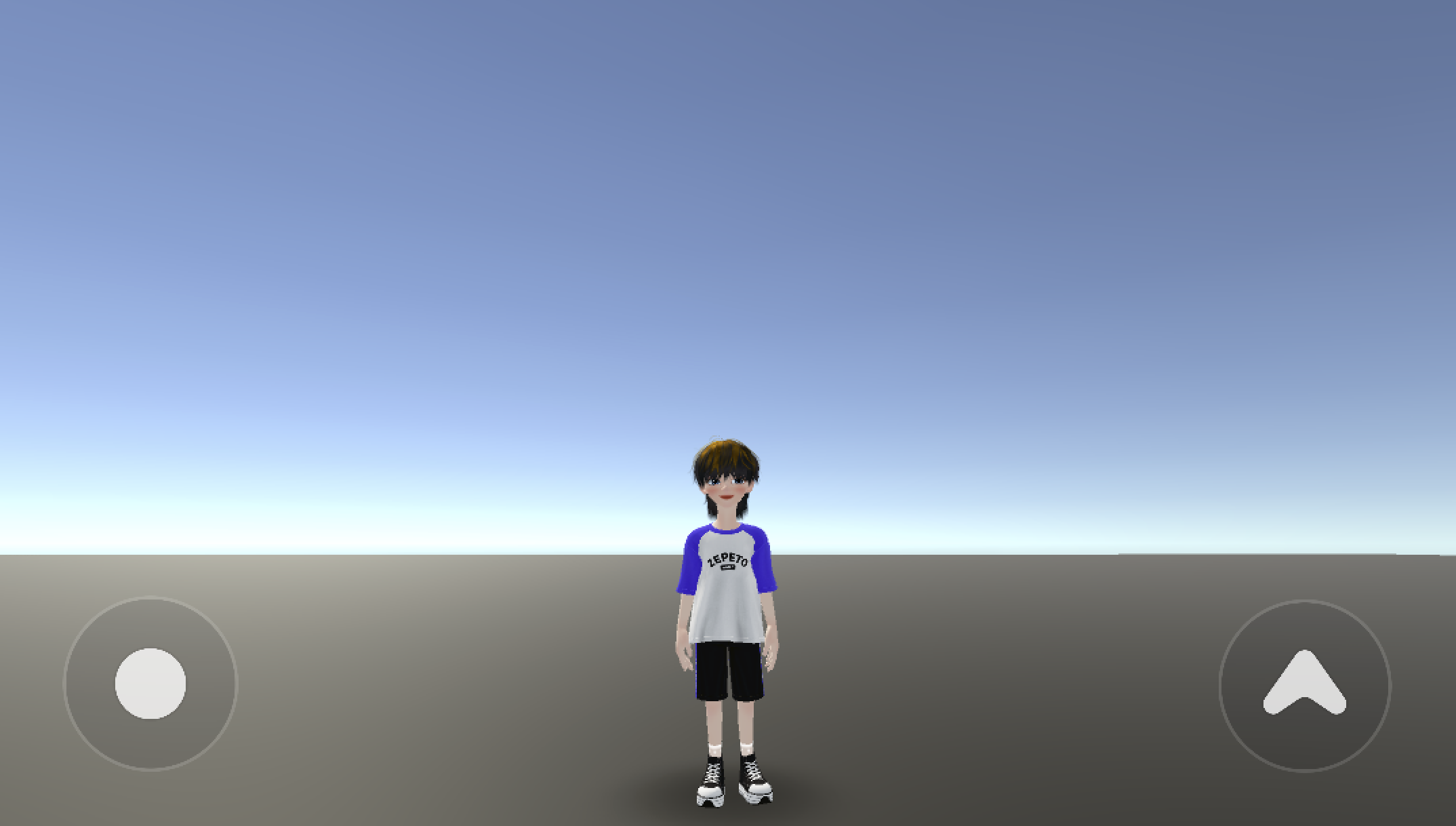
- The code below ensures that all players entering the world will have all their body modification items removed and will be dressed in the default ZEPETO costume.
- However, the multiplayer settings and basic server-client script implementation must be completed in advance.
ZepetoPlayers.instance.OnAddedPlayer.AddListener(() => {
ZepetoPlayers.instance.DeformDefaultCostume();
});
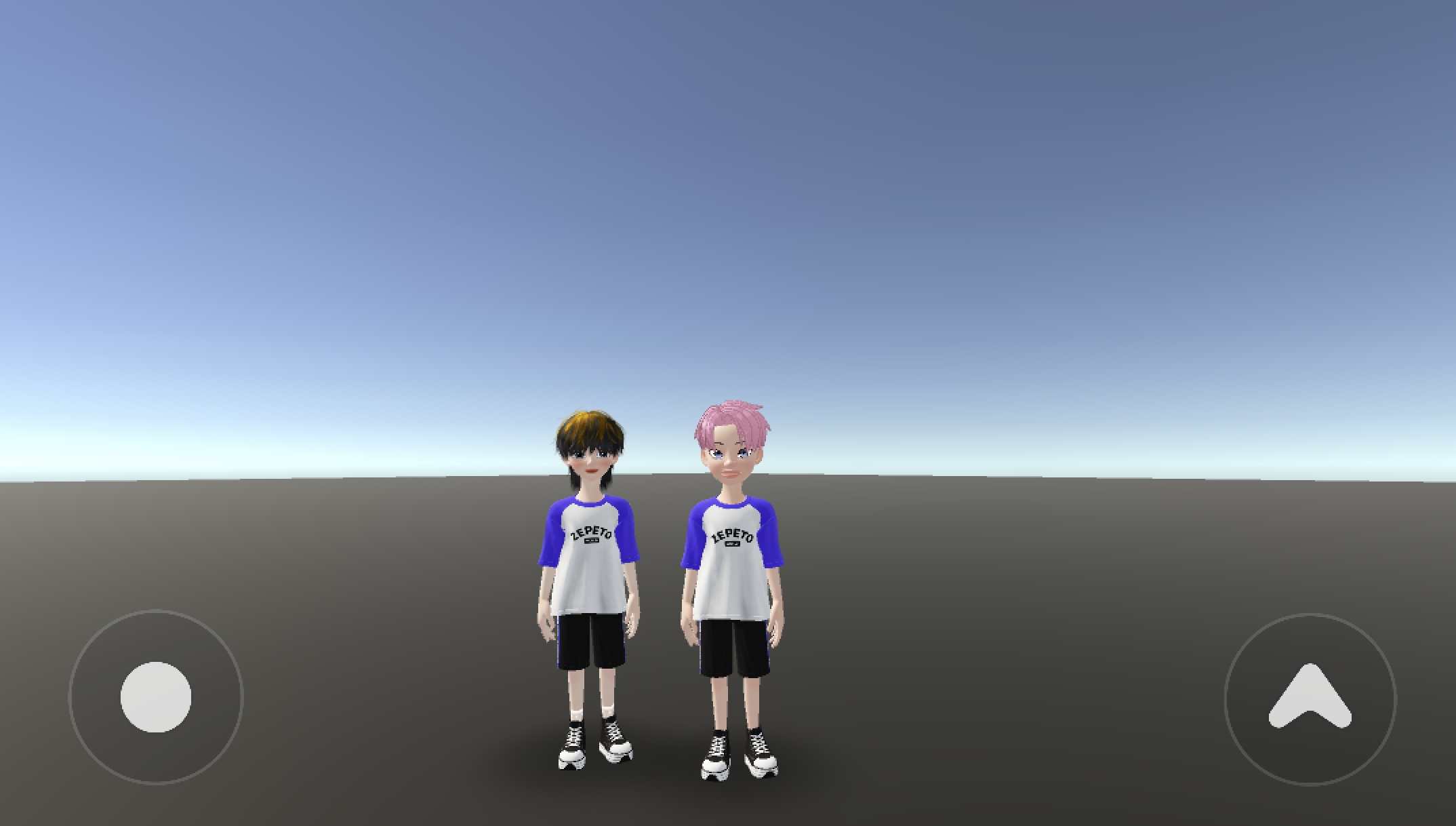
Caution
- Animated avatar faces are also classified as body modification items.
- Therefore, when using the
DeformDefaultProperties()
,DeformDefaultCostume()
APIs, please note that the appearance of animated avatars entering the world will also change.