You can use the ZEPETO.Module.Shop API to retrieve the item information of the currently connected user by category.
Install
Window → Package Manager → Please install ZEPETO.World Package 1.21.14 version or higher first.
Then install the ZEPETO.Module package 1.0.7 version or higher.
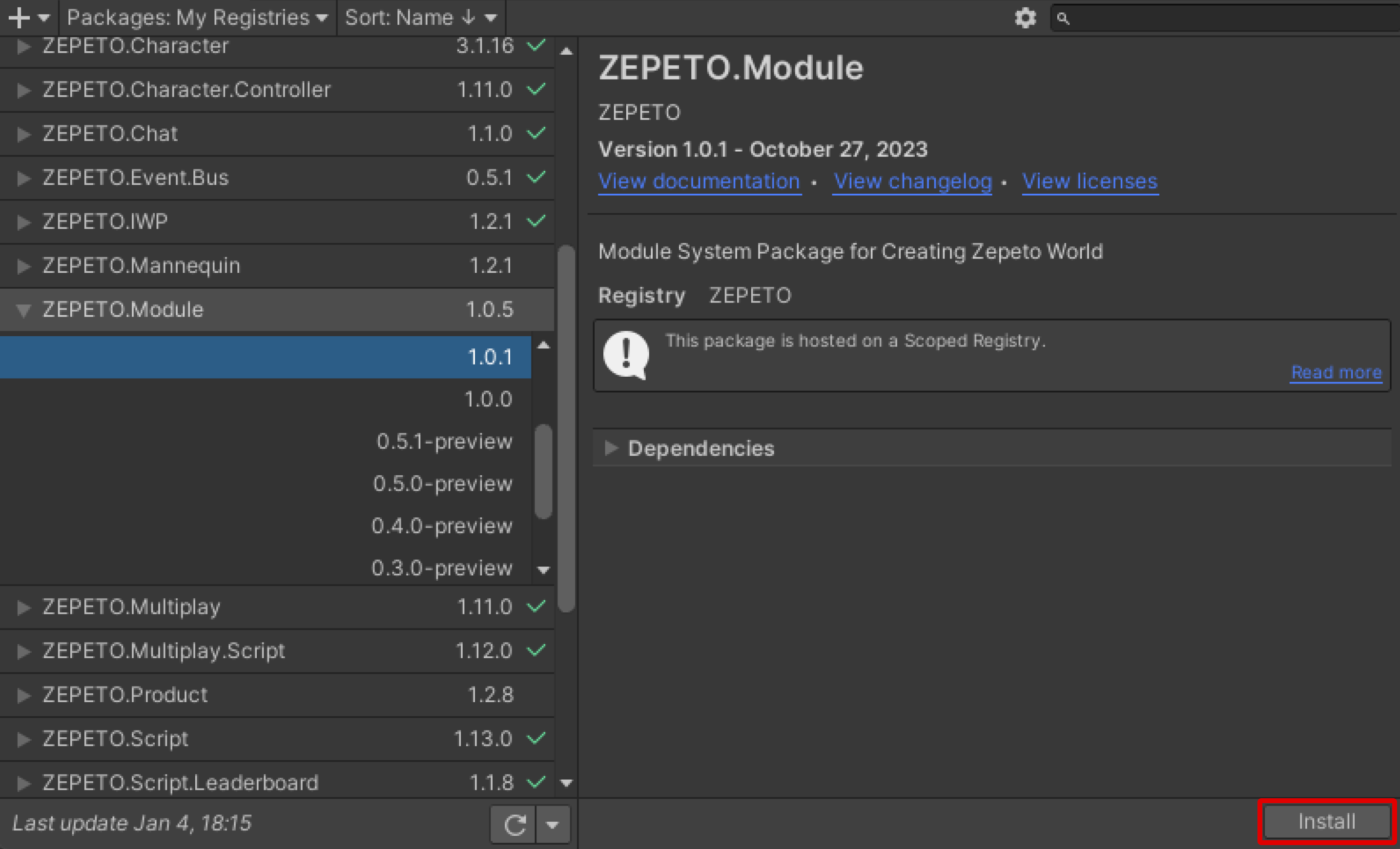
ZEPETO.Module.Shop API
If you're interested in the ZEPETO.Module.Shop API, refer to the documentation:
Please refer to the following guide. [ZEPETO.Module.Shop API]
Outputting Item Information Example
- Project > Create > ZEPETO > TypeScript is created and the name is changed to MyItemList.
- Write a sample script as shown below.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script'
import { ShopService, Category, ItemKeyword } from 'ZEPETO.Module.Shop'
import { ZepetoPropertyFlag } from 'Zepeto'
import { Texture2D, WaitUntil } from 'UnityEngine'
import { RawImage } from 'UnityEngine.UI'
import { Item } from 'ZEPETO.Module.Content'
export default class MyItemList extends ZepetoScriptBehaviour {
public thumbnailImage: RawImage;
Start() {
this.StartCoroutine(this.CoGetMyCategory());
}
*CoGetMyCategory() {
var requestCategory = ShopService.GetMyCategoryAsync();
yield new WaitUntil(() => requestCategory.keepWaiting == false);
if (requestCategory.responseData.isSuccess) {
let categoryInfo = requestCategory.responseData.category;
console.log(`[Category Info] ${categoryInfo.displayName} - length : ${categoryInfo.categories.Length}`);
let categories: Category[] = categoryInfo.categories;
for (const category of categories) {
console.log(`[Category displayName] ${category.displayName} `);
//Category Keyword is used as an argument in the ShopService.GetMyContentItemListAsync function
console.log(`[Category keyword] ${category.keyword} `);
}
}
this.StartCoroutine(this.CoGetMyItem());
}
*CoGetMyItem() {
var requestItemList = ShopService.GetMyContentItemListAsync(ItemKeyword.all, null);
yield new WaitUntil(() => requestItemList.keepWaiting == false);
if (requestItemList.responseData.isSuccess) {
let items: Item[] = requestItemList.responseData.items;
// The number of items received as a result of GetMyContentItemListAsync().
console.log(items.length);
for (let i = 0; i < items.length; ++i) {
const property: ZepetoPropertyFlag = items[i].property;
// Item ID and item property
console.log(`[Content Item] (id): ${items[i].id} | (property): ${property}`);
// Setting the item thumbnail as the texture of a rawImage
var textureReq = items[i].GetThumbnailAsync();
yield new WaitUntil(() => textureReq.keepWaiting == false);
let thumbnailTexture: Texture2D = textureReq.responseData.texture;
this.thumbnailImage.texture = thumbnailTexture;
}
}
}
}
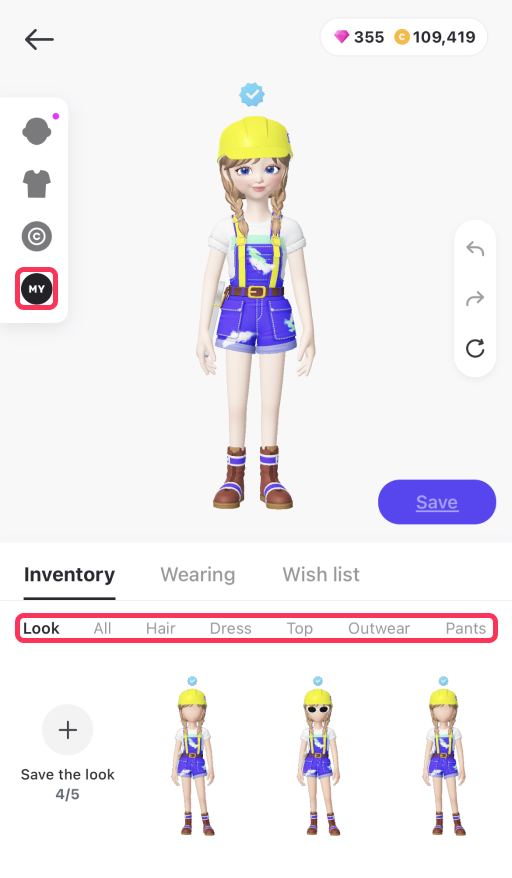
ZEPETO Shop > My > Inventory Category Information Example
- Script Description
- Retrieve My Item Tab Information
- Call ShopService.GetMyCategoryAsync() to know the item categories in the My Item tab.
- When you successfully receive a response, you can access the CategoryResponse object through the responseData property.
- CategoryResponse.category.categories is browsed and the item category list in the My Item tab is outputted.
- Retrieve Item List
- Call ShopService.GetMyContentItemListAsync(ItemKeyword.all, null) to retrieve the item list for all categories.
- You can choose to retrieve items from only certain categories. For instance, you can use ItemKeyword.hair instead of ItemKeyword.all to retrieve only the hair items.
- The second parameter is only used if you know the next page token to request. Set it to null the first time you call the API.
- For examples related to nextPageToken, check the information below.
- When you receive a successful response, you can access the ContentItemListResponse object through the responseData property
- ItemResponse.items is browsed and the ID and the part Enum code for each item is outputted.
- Also, the item thumbnail is set as the designated rawImage.
- Call ShopService.GetMyContentItemListAsync(ItemKeyword.all, null) to retrieve the item list for all categories.
- Retrieve My Item Tab Information
-
After you finish writing the script, add the script to the object in Scene.
-
Pressing the Play button will show you the category list of the My Item tab and the item information of all categories.
TIP
- If you create the item thumbnail prefab and have the thumbnails to be created dynamically based on the item list, you can display the item list thumbnail on the UI.
Example to Check Owned Status of User's Certain Item
You can utilize GetMyContentItemListAsync() to check if someone on a World is wearing particular clothing.
The following is a simple example to display a message on the UI of a user who owns a specific item.
- Project > Create > ZEPETO > TypeScript is created and the name is changed to CheckItem.
- Write a sample script as shown below.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script'
import { ShopService, ItemKeyword } from 'ZEPETO.Module.Shop'
import { ZepetoPropertyFlag } from 'Zepeto'
import { WaitUntil } from 'UnityEngine'
import { Text } from 'UnityEngine.UI'
import { Item } from 'ZEPETO.Module.Content'
export default class CheckItem extends ZepetoScriptBehaviour {
public resultText: Text;
public checkItemID: string;
Start() {
this.resultText.gameObject.SetActive(false);
this.StartCoroutine(this.CoCheckItem());
}
*CoCheckItem() {
let nextPageToken: string | null = null;
do {
// Request the list of the user's content items.
const requestItemList = ShopService.GetMyContentItemListAsync(ItemKeyword.all, nextPageToken);
yield new WaitUntil(() => requestItemList.keepWaiting == false);
if (requestItemList.responseData.isSuccess) {
// Get the content items from the response.
const contentItems: Item[] = requestItemList.responseData.items;
nextPageToken = requestItemList.responseData.nextPageToken;
// Iterate through the content items.
for (let i = 0; i < contentItems.length; ++i) {
const property: ZepetoPropertyFlag = contentItems[i].property;
// Check if the item ID matches the specified ID.
if (contentItems[i].id == this.checkItemID) {
// Activate the matched item message.
this.ActivateMatchedItem();
break;
}
}
}
else {
break;
}
} while (nextPageToken != null);
}
ActivateMatchedItem() {
// Show the matched item message.
this.resultText.gameObject.SetActive(true);
this.resultText.text = "Wow, you have an item I made!";
}
}
- Script Description
- This is a script that browses the 'My Item' list (all categories) of a user who is logged in to a World and finds the matching item ID entered in the Inspector.
- If there are many items, the nextPageToken value is not null. Therefore, the process is repeated with a do-while statement until the value becomes null.
- Notice how nextPageToken was provided as the second parameter of ShopService.GetMyContentItemListAsync(ItemKeyword.all, nextPageToken).
- When you receive a successful response, you can access the ContentItemListResponse object through the responseData property.
- ItemResponse.items is browsed to find a match for the entered item ID.
- If a matching item is found, ActivateMatchedItem() is called to display the text on the user's screen and end the loop.
-
After you finish writing the script, add the script to the object in Scene.
-
Enter the ID of the item you wish to check if a user in a World has on the Inspector.
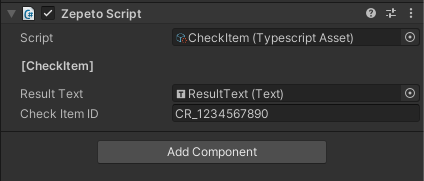
Item ID Input Example
- Press the Play button to run the process. Text will appear on the screen if the user logged into a World has an item that matches the entered item ID.
You can apply the example to create all sorts of interesting content.