Setting up a leaderboard
The leaderboard is a module that provides functions for saving and managing each player's rankings and high scores.
STEP 1 : Create a Leaderboard
To create a leaderboard, select the [Get LeaderboardInfo] button in ProjectSettings → Zepeto → ZepetoLeaderboard menu.
Select [Add Leaderboard] to add a new leaderboard.
If there is a leaderboard that already exists, the leaderboard will appear on the settings screen.
STEP 2 : Customize a Leaderboard
The pop up window shown below will appear when the [Add Leaderboard] button is selected. You will be able to adjust each parameter values.
Parameter | Description |
---|---|
Name | Leaderboard name |
Update Rule | Score update rules |
Max score | Record the user's highest score |
Min score | Record user's lowest score |
Accumulate score | Add user's scores and save as a record |
ResetInfo(s) | You can set a period for the scores to reset. - This is helpful if the leaderboard needs to be updated every season. - The scores will not be reset by default, but you can make adjustments by setting reset periods (monthly, weekly, daily). |
STEP 3 : Set score reset
Score reset rules are as follows:
Period | Description |
---|---|
None | Scores will not reset |
Day | Scores will reset daily at the set time (ex. daily ranking) |
Week | Scores will reset weekly on the set date and time (ex: weekly ranking) |
Month | Scores will reset monthly on the set date and time (ex: monthly ranking; the max Day value is 31, but if the month does not have 31 days, it will automatically adjust to the last day of the month) |
STEP 4 : Leaderboard ID
To use ZEPETOScript and leaderboard, you must have the Leaderboard ID information.
To access the Leaderboard information, you can go to Settings (under Edit in the Project Settings menu, go to Zepeto → Zepeto Leaderboard → Edit), or load from ZEPETOScript api.
Please refer to the following guide. [Searching Leaderboard Details]
Caution
- Once the reset rule is set, the leaderboard cannot be modified.
- To change the Reset Rule, you must create a new leaderboard.
- Reset time is based on UTC+0. Offset will be provided so that the time zone can be changed in the future.
Recording Score
LeaderboardAPI.SetScore() is an API that records the user's score on the leaderboard.
Leaderboard ID, Score value, the point of completion and the point of error are passed as an argument.
User information for the score will automatically be saed.
The following is an example for loading the LeaderboardAPI.SetScore function.
LeaderboardAPI.SetScore(this.leaderBoardId, this.score, this.OnResult, this.OnError);
The following is the code template for retrieving the user's score to the leaderboard.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { SetScoreResponse, LeaderboardAPI } from 'ZEPETO.Script.Leaderboard';
export default class SetScoreExample extends ZepetoScriptBehaviour {
public leaderboardId: string;
public score: number;
Start() {
LeaderboardAPI.SetScore(this.leaderboardId, this.score, this.OnResult, this.OnError);
}
OnResult(result: SetScoreResponse) {
console.log(`result.isSuccess: ${result.isSuccess}`);
}
OnError(error: string) {
console.error(error);
}
}
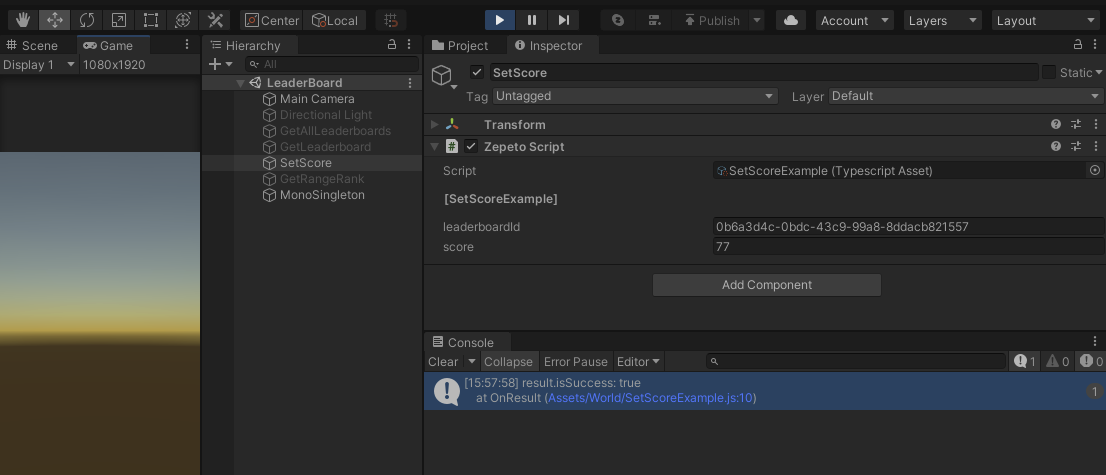
LeaderboardAPI.SetScore Run Screen
Searching Ranking Details
Get ranking information in a specific range
Use the LeaderboardAPI.GetRangeRank() function to load a specific range of ranking details.
The Boolean value to retrieve leaderboard ID, start ranking, last ranking, reset rule and last season's ranking information, and the callback function from the time of completion and time of error occurrence is passed as an argument.
User information that inquires ranking information is automatically entered, and up to 100 ranking information can be processed per inquiry (ex: paging in 1~100, 101~200 format for implementation)
Caution
If more than 100 data are loaded at a time, it may not work properly due to API load issues and rate-limits.
Example function call
An example of calling LeaderboardAPI.GetRangeRank() function is as follows:
LeaderboardAPI.GetRangeRank(this.leaderboardId, this.startRank, this.endRank, this.resetRule, false, this.OnResult, this.OnError);
The following code is an example for loading a specific range of ranking information.
You can use the Member value from OnResult to load the profile photo.
Please refer to the following guide. [User Information]
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { GetRangeRankResponse, LeaderboardAPI, ResetRule } from 'ZEPETO.Script.Leaderboard';
export default class GetRangeRankExample extends ZepetoScriptBehaviour {
public leaderboardId: string;
public startRank: number;
public endRank: number;
public resetRule: ResetRule;
Start() {
LeaderboardAPI.GetRangeRank(this.leaderboardId, this.startRank, this.endRank, this.resetRule, false,
this.OnResult, this.OnError);
}
OnResult(result: GetRangeRankResponse) {
console.log(`result.isSuccess: ${result.isSuccess}`);
if (result.rankInfo.myRank) {
console.log(`member: ${result.rankInfo.myRank.member}, rank: ${result.rankInfo.myRank.rank},
score: ${result.rankInfo.myRank.score}, name: ${result.rankInfo.myRank.name}`);
}
if (result.rankInfo.rankList) {
for (let i = 0; i < result.rankInfo.rankList.length; ++i) {
const rank = result.rankInfo.rankList.get_Item(i);
console.log(`i: ${i}, member: ${rank.member}, rank: ${rank.rank}, score: ${rank.score}, name: ${rank.name}`);
}
}
}
OnError(error: string) {
console.error(error);
}
}
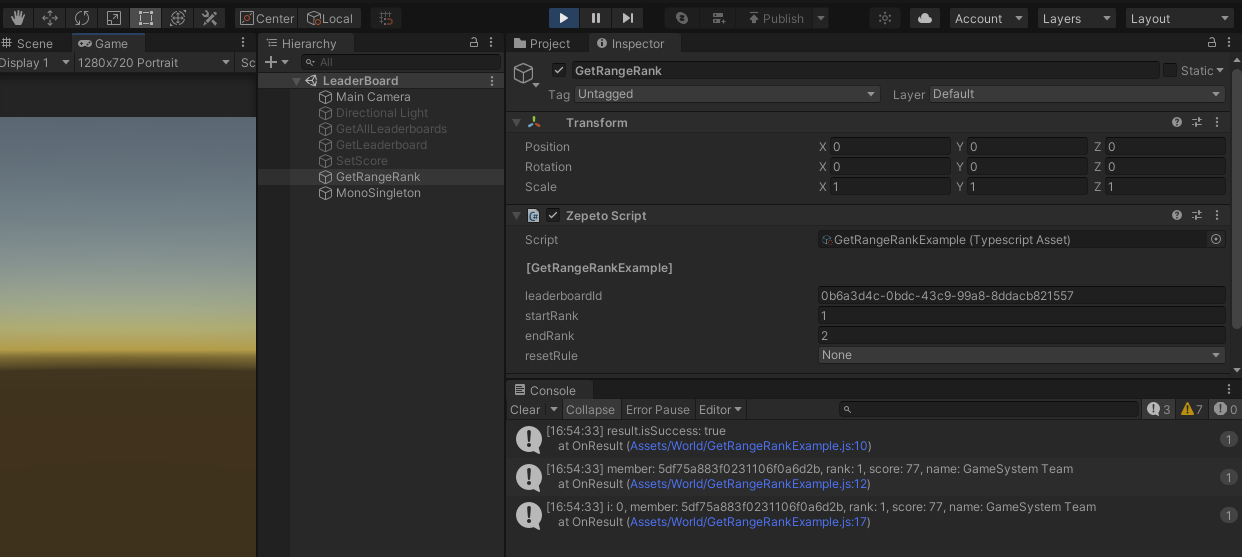
LeaderboardAPI.GetRangeRank Run Screen
Searching Leaderboard Details
Search All Leaderboards
Use the LeaderboardAPI.GetAllLeaderboards function to load all leaderboard information in the specified World.
The callback function from the time of completion to the time of error occurrence is passed as an argument.
An example of loading LeaderboardAPI.GetAllLeaderboards function is as follows:
LeaderboardAPI.GetAllLeaderboards(this.OnResult, this.OnError);
The following is an example for loading the entire leaderboard information.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { GetAllLeaderboardsResponse, LeaderboardAPI } from 'ZEPETO.Script.Leaderboard';
export default class GetAllLeaderboardExample extends ZepetoScriptBehaviour {
Start() {
LeaderboardAPI.GetAllLeaderboards(this.OnResult, this.OnError);
}
OnResult(result: GetAllLeaderboardsResponse) {
console.log(`result.isSuccess: ${result.isSuccess}`);
if (result.leaderboards) {
for (let i = 0; i < result.leaderboards.length; ++i) {
const leaderboard = result.leaderboards[i];
console.log(`i: ${i}, id: ${leaderboard.id}, name: ${leaderboard.name}`);
}
}
}
OnError(error: string) {
console.error(error);
}
}
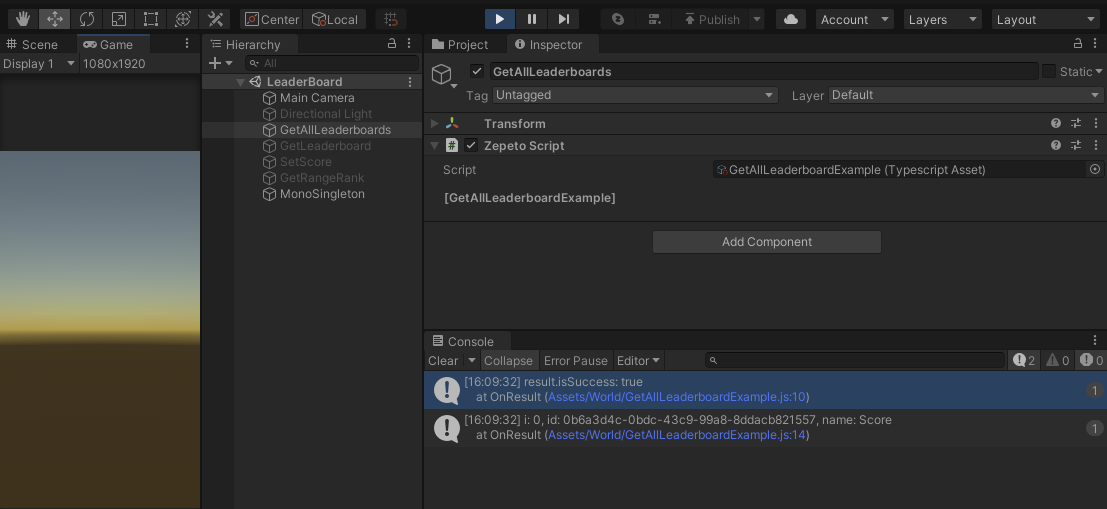
LeaderboardAPI.GetAllLeaderboards Run Screen
Search Specific Leaderboards
Use the LeaderboardAPI.GetLeaderboardsfunction to load specific leaderboard information.
The callback function for the leaderboard ID, the time of completion, the time of error occurrence are passed as an argument.
An example of loading LeaderboardAPI.GetLeaderboard function is as follows:
LeaderboardAPI.GetLeaderboard(this.leaderboardId, this.OnResult, this.OnError);
The following is an example for loading certain leaderboard inofrmation.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { GetLeaderboardResponse, LeaderboardAPI } from 'ZEPETO.Script.Leaderboard';
export default class GetLeaderboardExample extends ZepetoScriptBehaviour {
public leaderboardId: string;
Start() {
LeaderboardAPI.GetLeaderboard(this.leaderboardId, this.OnResult, this.OnError);
}
OnResult(result: GetLeaderboardResponse) {
console.log(`result.isSuccess: ${result.isSuccess}`);
if (result.leaderboard) {
console.log(`id: ${result.leaderboard.id}, name: ${result.leaderboard.name}`);
}
}
OnError(error: string) {
console.error(error);
}
}
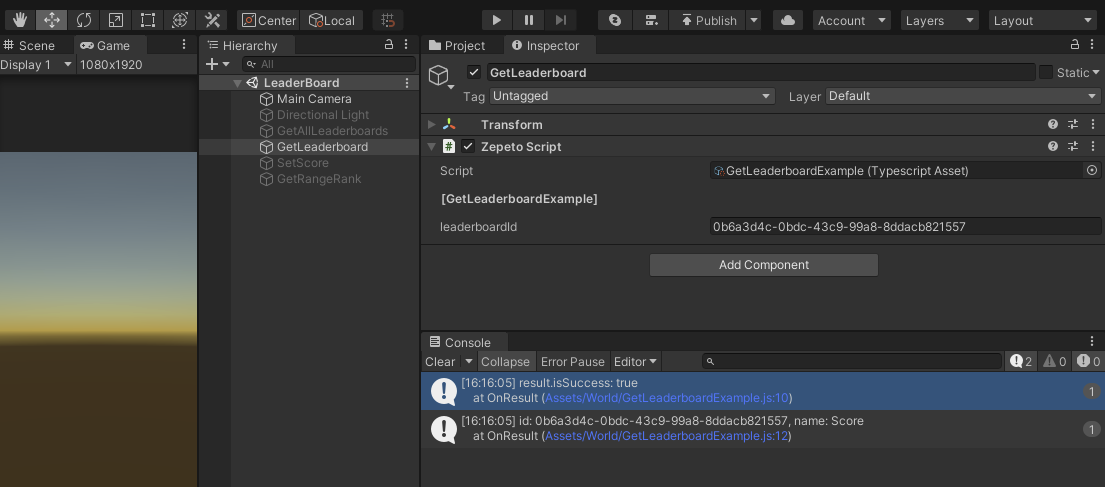
LeaderboardAPI.GetLeaderboard Run Screen
Leaderboard Usage Example
- The following is an example of a leaderboard applied to the official ZEPETO World Slime Party.
- By setting, reading, and utilizing API calls to fetch rankings within a specific range, you can structure it as follows in the UI.
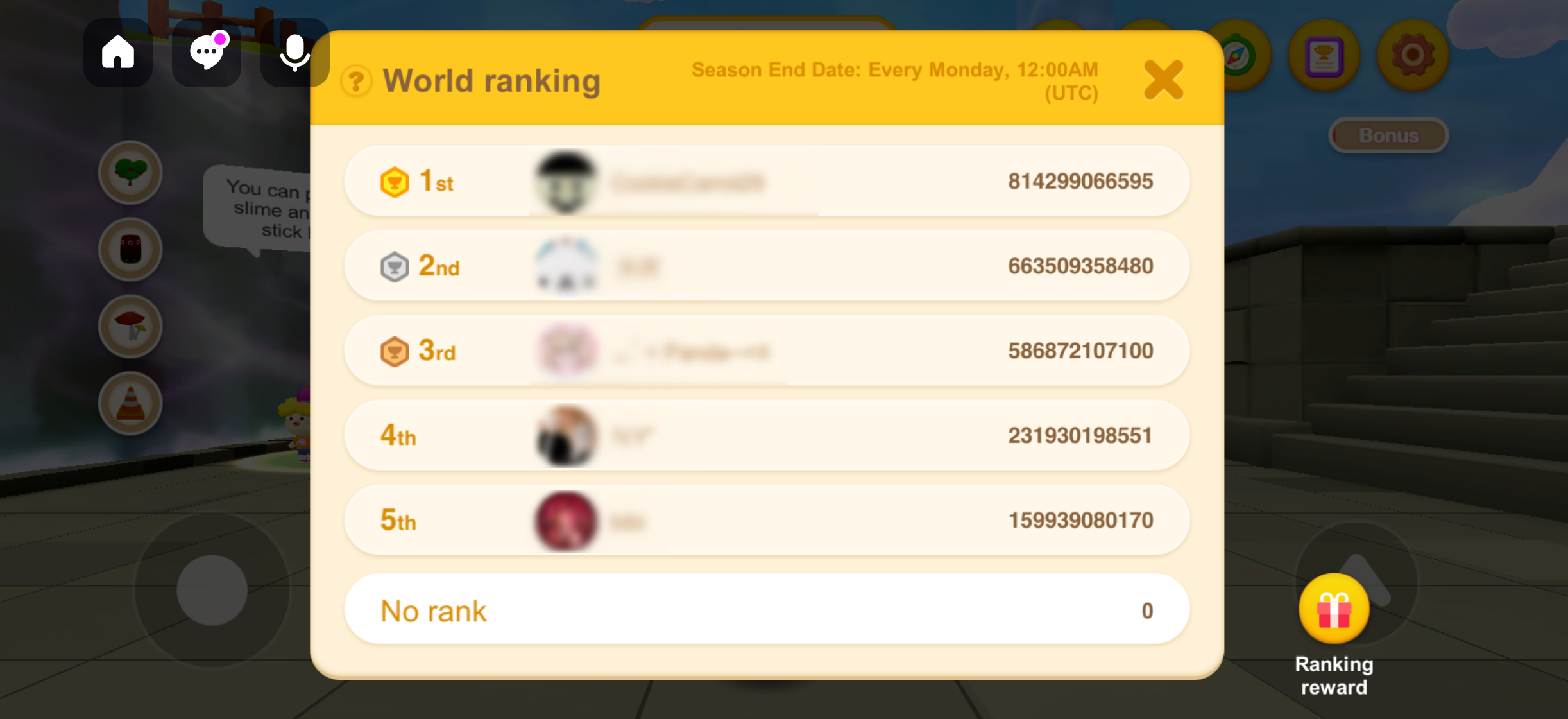