How do you use a Singleton?
A Singleton is a design pattern that ensures there is only one instance of a particular class within a program.
The Singleton pattern provides a single global instance of a class, making it easily accessible from other scripts that need it, such as managing logic, audio, UI, and centralized resources within the world.
In ZEPETOScript, you can implement a Singleton in the following way:
👍 Tips
- If there is already a GameObject with a Singleton script attached in the Scene, you can use this format.
- If there isn't one, add code to create the GameObject.
- Example: new GameObject("ObjectName").AddComponent<ClassName>();
Let's implement a Singleton for managing UI in an example:
1) Add UI > Text and Button to the Scene.
- Set up the logic to increase the score when the "IncreaseScore" button is pressed
- Set up the logic to decrease the score when the "DecreaseScore" button is pressed

2) Create a script called "ScoreManager," where the Singleton will be applied.
- The ScoreManager script will be responsible for increasing/decreasing the score and updating the Text when called from other scripts.
- Script Description
- private static _instance: This is a private static variable within the class, initially set to null.
- public static get Instance: This static property provides a way for external code to access the unique instance of this class.
- Check if (this.\_instance == null). If it’s null, use GameObject.FindObjectOfType<>() to find and return a component of type ClassName, and assign it to the static variable.
3) After writing the script, return to the Inspector and connect the Text component.

4) Next, create a script called "ScoreLogicSample" to demonstrate how to call the Singleton. Ensure that ScoreManager and ScoreLogicSample are in the same directory.

- Write code like this:
- It's important to specify the path correctly when getting the Singleton script.
- You can adapt the part where ScoreManager is called in your own script as needed.
5) Press the [▶︎(play)] button to test.
- You should observe the functions in ScoreManager being called when you click the buttons in ScoreLogicSample, making it work as expected.
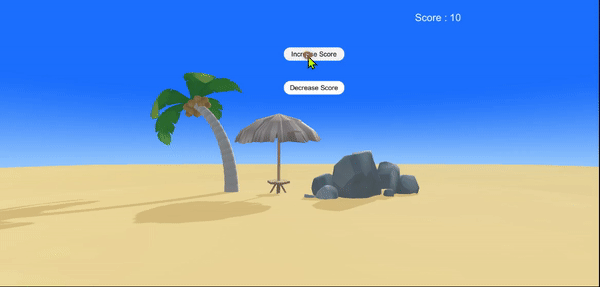
